The CSS pseudo-class :indeterminate
is a handy tool that can add a layer of sophistication to user interface interactions. Primarily, it helps to indicate an intermediate state in UI elements, such as a checkbox, when a user’s selection is partially complete. So, let’s examine a straightforward example that showcases the potential application of this feature.
Your Web Designer Toolbox
Unlimited Downloads: 500,000+ Web Templates, Icon Sets, Themes & Design Assets
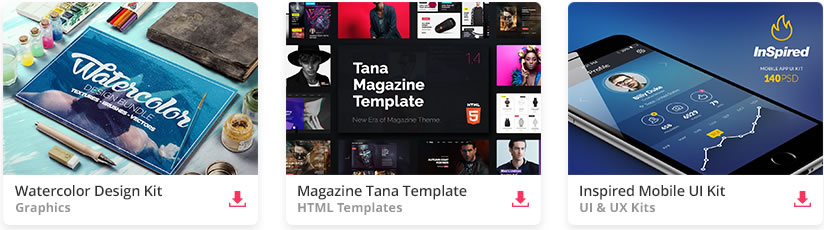
Setting Up the HTML Structure
<div class="container"> <ul> <li> <input type="checkbox" id="category"> <label for="category"> Groceries </label> <ul> <li> <label> <input type="checkbox" class="subCategory"> Fruits </label> </li> <li> <label> <input type="checkbox" class="subCategory"> Vegetables </label> </li> <li> <label> <input type="checkbox" class="subCategory"> Dairy </label> </li> </ul> </li> </ul> </div>
In this scenario, we’ve structured a list with a main checkbox labeled “Groceries” and three sub-categories.
Enhancing Visual Feedback with CSS
Next, we focus on using CSS to visually distinguish between various states of our checkboxes.
body { color: #555; font-size: 1.25em; font-family: system-ui; } ul { list-style: none; } .container { margin: 40px auto; max-width: 700px; } li { margin-top: 1em; } label { font-weight: bold; } input[type="checkbox"]:indeterminate + label { color: #f39c12; }
The input[type="checkbox"]:indeterminate + label
selector is key here. It targets the label of the main checkbox when it’s in the indeterminate state, changing its color to indicate partial selection. The rest of the CSS provides general aesthetic tweaks.
Introducing Interactivity with JavaScript
const checkAll = document.getElementById('category'); const checkboxes = document.querySelectorAll('input.subCategory'); checkboxes.forEach(checkbox = >{ checkbox.addEventListener('click', () = >{ const checkedCount = document.querySelectorAll('input.subCategory:checked').length; checkAll.checked = checkedCount > 0; checkAll.indeterminate = checkedCount > 0 && checkedCount < checkboxes.length; }); }); checkAll.addEventListener('click', () = >{ checkboxes.forEach(checkbox = >{ checkbox.checked = checkAll.checked; }); });
The JavaScript code here manages the state of the main checkbox based on the sub-options selected. The main checkbox enters an intermediate state, displaying a horizontal line as styled by Chrome on Windows when some sub-options are chosen. When all the sub-options are checked, the main checkbox returns to its original color and enters the checked state.
The Final Result
As each grocery item is selected, the main “Groceries” checkbox alternates between states, reflecting the selection status of the sub-items. This creates a clear visual cue to the user about the selection status.
Our demonstration through this HTML, CSS, and JavaScript blend, is just one of many tools you can use to enhance UI clarity. Don’t stop here—consider how other CSS pseudo-classes like :hover
, :focus
, or :active
can also be utilized to provide real-time feedback to users. As you expand your web design toolkit, remember the goal is to create user experiences that are not only visually appealing but also communicate effectively with your audience.
This post may contain affiliate links. See our disclosure about affiliate links here.